前回作ったUDP通信コンポーネントの覚書として、簡単なチャットソフトを作ってみた
前回はUDP通信コンポーネントを作りツールボックスへ登録した訳だが、それを使って今度は簡単なチャットソフトを作ってみよう。
作り方ですが、新しいプロジェクトの作成でWindowsフォームアプリケーション(.NETFramework) C#を選んで新規プロジェクトを作成してください。
出来たフォームに以下のようにコントロールを配置します。
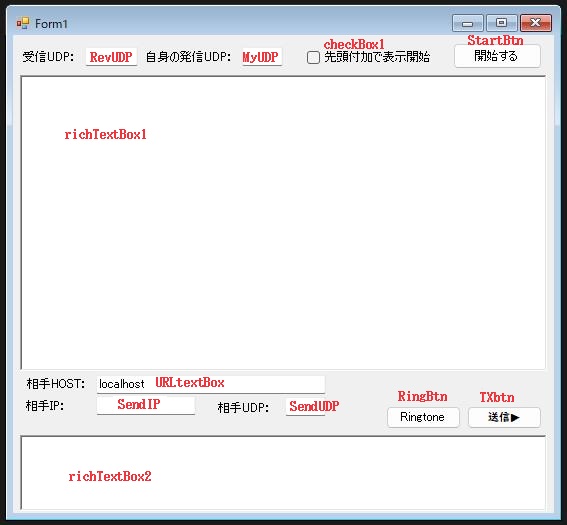
コントロール名は何でも良いのですが説明の都合上以下のように命名します。
RichTextBoxがふたつ、CheckBoxをひとつ、特に名前はそのままで配置。
ボタンは3つ、StartBtnとTXbtnとRingBtnという名前に変更します。
TextBoxは5つ用意します。名前はそれぞれRevUDP,MyUDP,URLtextBox,SendIP,SendUDP。
このフォームに前回作成したUDP通信コントロールをUDPCompo1という名前で配置します。
後は実際のコードを見れば理解し易いと思うのでそのまま記載します。
using System;
using System.Drawing;
using System.Net.Sockets;
using System.Net;
using System.Windows.Forms;
namespace WindowsFormsApp3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
//UDPポート設定 環境に合わせて変更しよう
MyUDP.Text = "2030";
RevUDP.Text = "2020";
SendUDP.Text = "2020";
// 見本用初期HOST URL
URLtextBox.Text = "localhost";
// URL→IP変換
SendIP.Text = ConvertIP(URLtextBox.Text);
}
/// <summary>
/// HOST名をIPAdressに変換します UDPCompoではIPAdressでの指定が必要です
/// </summary>
/// <param name="host"></param>
/// <returns>adress文字列</returns>
private string ConvertIP(string host)
{
try
{
string adr = "";
IPHostEntry ip = Dns.GetHostEntry(host);
foreach (IPAddress address in ip.AddressList)
{
if (address.AddressFamily == AddressFamily.InterNetwork)
adr = address.ToString();
}
return adr;
}
catch
{
return "正しくないホスト名";
}
}
/// <summary>
/// 正しいIPかチェック
/// </summary>
/// <param name="IPStr"></param>
/// <returns>正true, 非false</returns>
private bool VerifyIP(string IPStr)
{
string ipstr = SendIP.Text;
IPAddress ipaddr;
return IPAddress.TryParse(ipstr, out ipaddr);
}
private void SendText()
{
//! テキストボックスから、送信するテキストを取り出す.
String strSend = richTextBox2.Text;
//! 送信するテキストがない場合、データ送信は行わない.
if (string.IsNullOrEmpty(strSend) == true) return;
// 開始されていないなら
if (UDPCompo1.udpForReceive == null)
{
MessageBox.Show("まだ開始ボタンが押されていません!!", "確認!");
return;
}
// IPチェック
if (VerifyIP(SendIP.Text))
{
// 送信パラメーター(相手IP、自分UDP、相手UDP、送信テキストデータ)
UDPCompo1.Send(SendIP.Text, int.Parse(MyUDP.Text), int.Parse(SendUDP.Text), strSend);
// 送信したので入力をクリアし次の入力を待つ.
richTextBox2.Clear();
richTextBox2.Focus();
}
else
{
MessageBox.Show("IPが正しくありません!!", "確認!");
}
}
private void StartBtn_Click(object sender, EventArgs e)
{
if (UDPCompo1.udpForReceive != null)
{
//通信中なので停止
UDPCompo1.udpForReceive.Close();
UDPCompo1.udpForReceive = null;
StartBtn.BackColor = SystemColors.Control;
StartBtn.Text = "開始する";
StartBtn.ForeColor = SystemColors.ControlText;
}
else
{
//停止しているので開始
StartBtn.BackColor = SystemColors.GradientActiveCaption;
StartBtn.Text = "受信停止する";
StartBtn.ForeColor = Color.Red;
// MyUDP初期化 (ref RichTextBox,追加モード,IPアドレス,送信ポート,宛先ポート,受信ポート)
if (checkBox1.Checked)
{
UDPCompo1.Init(ref richTextBox1, ref richTextBox2, false, int.Parse(RevUDP.Text));
}
else
{
UDPCompo1.Init(ref richTextBox1, ref richTextBox2, true, int.Parse(RevUDP.Text));
}
}
ActiveControl = richTextBox2;
}
private void TextBox_KeyPress(object sender, KeyPressEventArgs e)
{
//EnterやEscapeキーでビープ音が鳴らないようにする
//すべてのテキストボックスのKeyPressにこれを指定しましょう
if (e.KeyChar == (char)Keys.Enter || e.KeyChar == (char)Keys.Escape)
{
e.Handled = true;
}
}
private void URLtextBox_Leave(object sender, EventArgs e)
{
SendIP.Text = ConvertIP(URLtextBox.Text);
}
private void richTextBox2_PreviewKeyDown(object sender, PreviewKeyDownEventArgs e)
{
if (e.KeyCode == Keys.Enter)
{
if (e.Shift)
{
//shift + Enterのときは送信
SendText();
}
// ただのEnterは改行するので何もしない
}
}
private void checkBox1_CheckedChanged(object sender, EventArgs e)
{
if (UDPCompo1.udpForReceive != null)
{
//通信中なので停止
UDPCompo1.udpForReceive.Close();
UDPCompo1.udpForReceive = null;
}
//表示変更
StartBtn.BackColor = SystemColors.GradientActiveCaption;
StartBtn.Text = "受信停止する";
StartBtn.ForeColor = Color.Red;
// MyUDP初期化 (ref 受信RichTextBox,ref 送信RichTextBox,追加モード,受信ポート)
if (checkBox1.Checked)
{
UDPCompo1.Init(ref richTextBox1, ref richTextBox2, false, int.Parse(RevUDP.Text));
}
else
{
UDPCompo1.Init(ref richTextBox1, ref richTextBox2, true, int.Parse(RevUDP.Text));
}
}
private void RingBtn_Click(object sender, EventArgs e)
{
// ”SND_LOGON”を送り 呼び出し音を鳴らす
UDPCompo1.Send(SendIP.Text, int.Parse(MyUDP.Text), int.Parse(SendUDP.Text), "SEND_LOGON");
UDPCompo1.ShowSoundOn();
}
private void TXbtn_Click(object sender, EventArgs e)
{
SendText();
}
}
UDPCompo1.Init(..)で開始すれば自分のUDP宛に届いたものはRichTextBoxに書き込まれます。
Initで指定するRichTextBox名は頭に必ずrefが必要なのでお忘れなく!!
送信はUDPCompo1.Send(相手IP、自分UDP、相手UDP、送信テキストデータ) で送ります。
相手のIPが正しく入力されているかのチェックをVerifyIPでチェックしてます。URLではそのまま送れないのでIPアドレスに変換もしています。
シンプルに記述も少なくて済むのでUDPコンポの使用の参考にどうぞ!!